3일차에서는 BOM, Event, DOM에 대해 배웠고
JSON과 Ajax에 대해서도 살펴보았습니다. 아마 내용은 분리되어 포스팅될 것 같습니다.
먼저, BOM이란 Browser Object Model을 뜻하며 웹 브라우저의 모든 객체들을 참조하는 브라우저 모델을 말합니다.
The Browser Object Model (BOM) is a browser-specific convention referring to all the objects exposed by the web browser. Unlike the Document Object Model, there is no standard for implementation and no strict definition, so browser vendors are free to implement the BOM in any way they wish.
Product software implementation method - Wikipedia
From Wikipedia, the free encyclopedia Jump to navigation Jump to search A product software implementation method is a systematically structured approach to effectively integrate a software based service or component into the workflow of an organizational s
en.wikipedia.org
브라우저를 실행하면 자동으로 생성되는 5가지 객체들이 있는데
Window, Screen, Navigator, History, Location 입니다.
Window : 브라우저 창을 의미
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
// Window 객체 (클래스) : 브라우저의 창 의미
/*
특징 : 1. 전역객체 (global 객체) 라고 부른다. (python : __builtins__)
2. Window 객체내의 요소를 사용시 정확한 코드는 window.변수 또는 window.메서드() 형식으로 사용해야 된다.
일반적으로 window 키워드를 생략해서 사용한다.
예> alert(), print(), parseInt(), prompt(), setTimeout(), setInterval(),
document, onclick, onXXX, history, screen, navigator, location,
opener <-- 자식 객체에서 자신을 생성해준 부모 객체를 참조할 때 사용
*/
console.log(window);
window.alert('AAA');
alert("BBB")
</script>
</head>
<body>
</body>
</html>
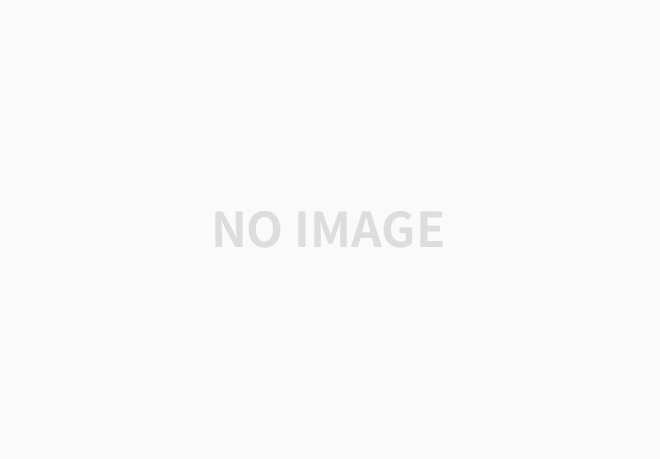
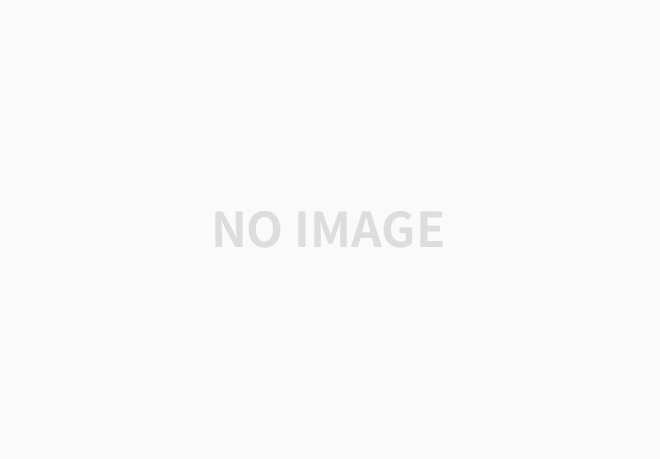
Window_parents
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
var childWin;
function child_open(){
childWin = window.open("Window_child.html", "childWin", "width=250, height =250");
}
function child_close(){
childWin.close();
}
function current_close(){
window.close();
}
</script>
</head>
<body>
<h2>Window_parents(부모창)</h2>
<button onclick = "child_open()">자식창 열기</button>
<button onclick = "child_close()">자식창 닫기</button>
<button onclick = "current_close()">현재창 닫기</button>
</body>
</html>
Window_child
opener : 자식 객체에서 자신을 생성해준 부모 객체를 참조할 때 사용한다.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
function parent_close(){
opener.close();
}
function current_close(){
window.close();
}
</script>
</head>
<body>
<h2>Window_child(자식창)</h2>
<button onclick = "parent_close()">부모창 닫기</button>
<button onclick = "current_close()">현재창 닫기</button>
</body>
</html>
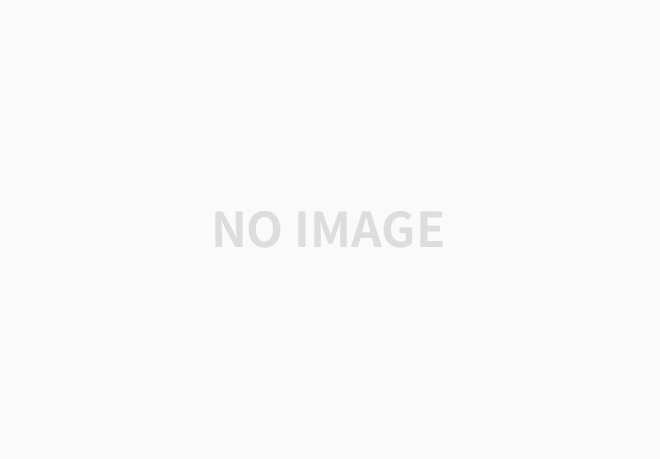
Screen : 브라우저 화면 정보
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
//Screen 객체 (클래스) : 브라우저 화면 정보
/* https://developer.mozilla.org/en-US/docs/Web/API/Screen */
console.log(window.screen);
console.log(screen);
console.log(screen.width);
console.log(screen.height);
</script>
</head>
<body>
</body>
</html>
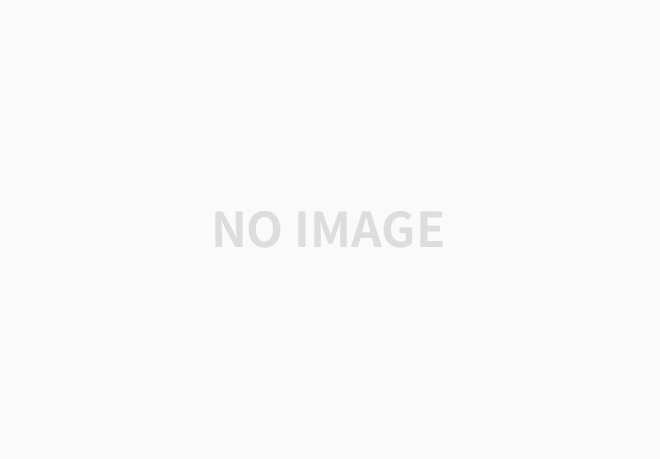
Navigator : 브라우저의 정보 (이름, 정보 등)
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
//Navigator 객체(클래스): 브라우저의 정보(이름, 버전 등)
console.log(navigator);
console.log(navigator.userAgent);
console.log(navigator.onLine);
</script>
</head>
<body>
</body>
</html>
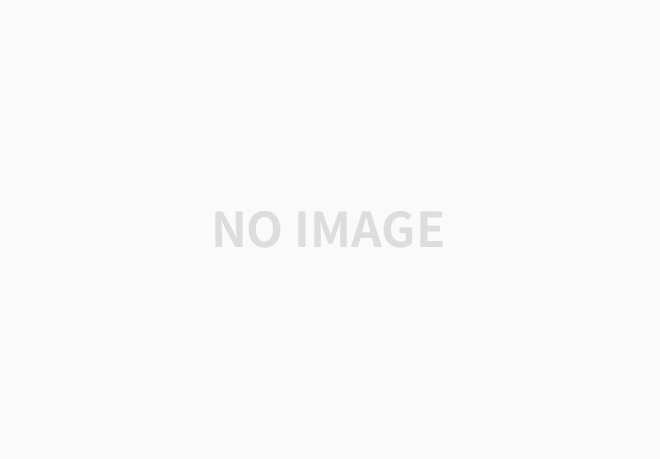
History : Session History를 조작하여 브라우저의 session 기록 내에서 앞/뒤로 url 이동
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
// History 객체(클래스) : session history를 조작하여 브라우저의 session 기록 내에서 앞/뒤로 url 이동
/* https://developer.mozilla.org/en-US/docs/Web/API/History */
console.log(history)
</script>
</head>
<body>
<h1>첫화면</h1>
<a href="history2.html">두번째 화면</a>
</body>
</html>
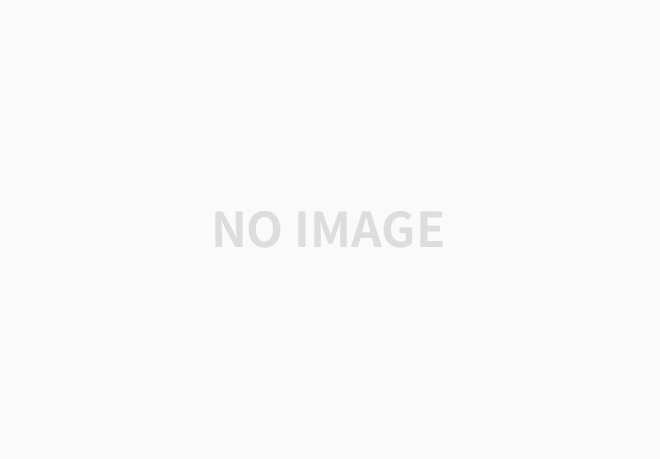
history2
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
// History 객체(클래스) : 브라우저의 앞/뒤
console.log(history)
function h1(){
history.back();
}
function h3(){
history.forward();
}
</script>
</head>
<body>
<h1>두번째화면(history2.html)</h1>
<a href="history3.html">세번째 화면</a>
<button onclick = "h1()">이전화면</button>
<button onclick = "h3()">다음화면</button>
</body>
</html>
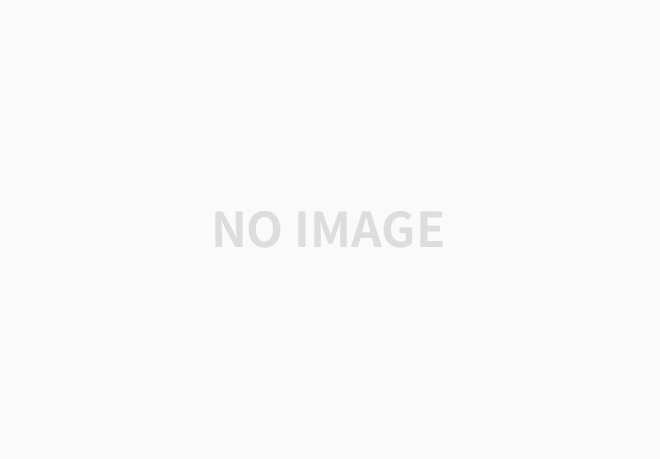
history3
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
// History 객체(클래스) : 브라우저의 앞/뒤
console.log(history)
function h2(){
history.back();
}
function h1(){
history.go(-2);
}
</script>
</head>
<body>
<h1>세번째화면(history3.html)</h1>
<a href="javascript:h2()">이전화면(a태그)</a>
<button onclick = "h2()">이전화면(click버튼)</button>
<button onclick = "h1()">첫 화면(onclick버튼)</button>
</body>
</html>
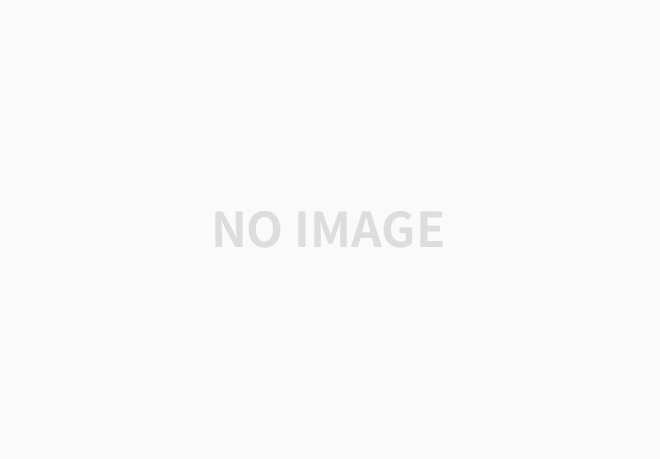
Location : 브라우저의 url
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script type="text/javascript">
//Location : 브라우저 url
console.log(location);
function a(){
location.href="http://www.daum.net";
}
function b(){
location.href="http://www.google.com";
}
function c(){
location.reload();
}
</script>
</head>
<body>
<button onclick = "a()">Daum</button>
<button onclick = "b()">Google</button>
<button onclick = "c()">새로고침</button>
</body>
</html>
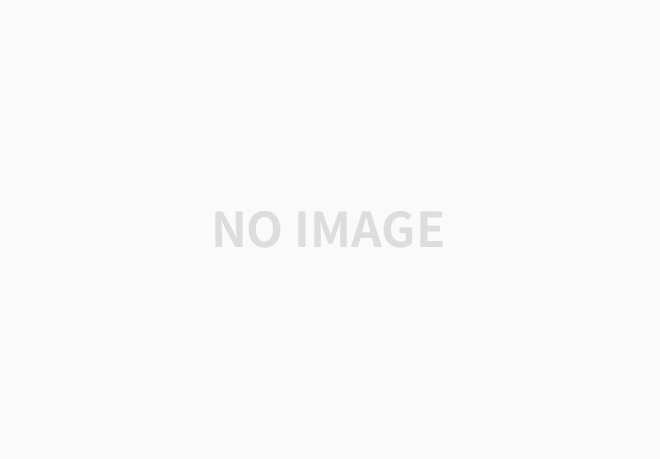
'SK 행복성장캠퍼스 > Java Script' 카테고리의 다른 글
2020-09-21,JavaScript_3일차 (Event, DOM) (0) | 2020.10.05 |
---|---|
2020-09-18,JavaScript_2일차(2) (0) | 2020.10.04 |
2020-09-18,JavaScript_2일차 (0) | 2020.09.28 |
2020-09-17,JavaScript_1일차 (0) | 2020.09.27 |
댓글